Integrating Plaid into Your Flutter App: A Step-by-Step Guide
- Arpan Desai
- Jan 24
- 5 min read
Updated: Feb 20
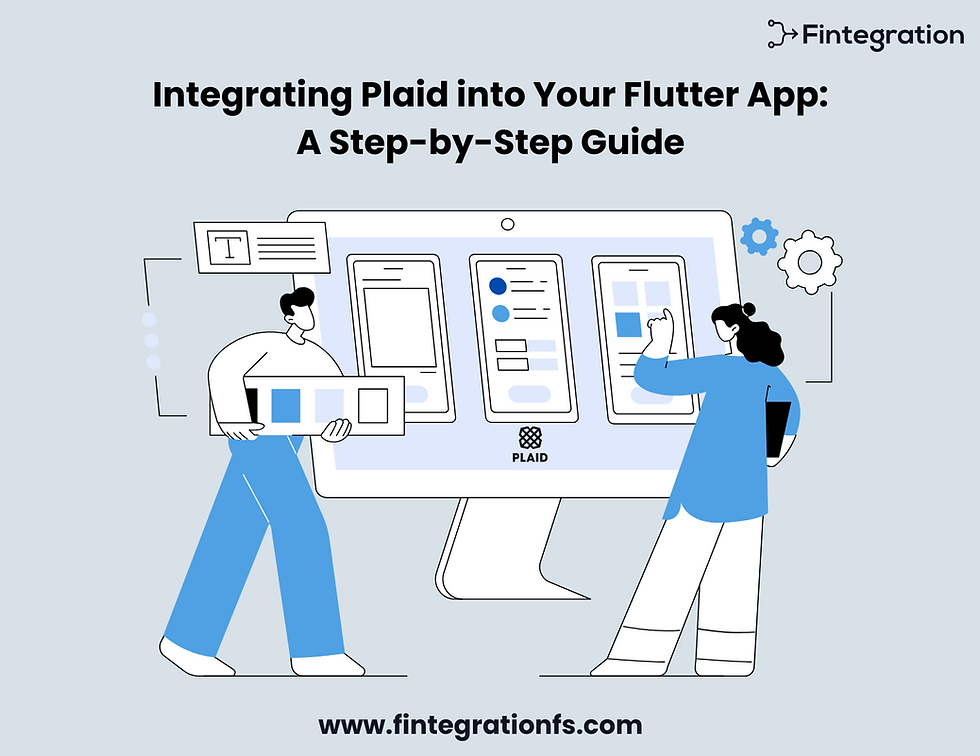
Introduction
A brief overview of Plaid and its importance in the fintech ecosystem.
Explanation of the benefits of integrating Plaid into a Flutter app.
Outline the goal: successfully implementing Plaid API for financial data access in Flutter.
Understanding Plaid
Provide an overview of what Plaid is and how it works.
Discussion on the types of API endpoints provided by Plaid.
Explanation of use cases for Plaid in apps (e.g., bank account linking, transaction retrieval).
Setting Up Your Development Environment
Step-by-step instructions for preparing the development environment.
Requirements for using Plaid Flutter and integration with Plaid.
Installation of necessary dependencies and tools (e.g., Flutter SDK, libraries).
Creating a New Plaid Flutter Project
Guidance on setting up a new Plaid Flutter project.
Configuring project settings for API integration.
Suggested folder structure for organizing files and code.
Implementing Plaid in Your Flutter App
Step 1: Adding Plaid Dependencies
How to add Plaid SDK to your Flutter application.
Managing dependencies in `pubspec.yaml`.
Step 2: Authentication with Plaid
Instructions for creating a Plaid account and application.
Utilizing Plaid Link for user authentication.
Setting up environment variables for secure credential handling.
Step 3: Integrating Plaid Link
Detailed process of embedding Plaid Link into your Flutter app.
Explanation of customization options for the Plaid Link UI.
Handling the success and error events during the Link process.
Retrieving Financial Data
Accessing Transactions
Methods to retrieve user transaction data via Plaid API.
Structuring API calls and managing responses in your app.
Account Information Access
Steps to pull account balance and details.
Parsing and displaying account information in Plaid Flutter.
Error Handling and Troubleshooting
Common issues faced during integration and solutions.
Best practices for handling API errors gracefully.
Resources for troubleshooting (e.g., Plaid documentation, community forums).
Testing Your Integration
Importance of testing API integrations thoroughly.
Strategies for unit and integration testing.
Using mock services to simulate Plaid responses.
Deployment Considerations
Key points to consider when deploying a Plaid Flutter app with Plaid integration.
Ensuring compliance with data security and privacy regulations.
Monitoring API usage and performance post-deployment.
Conclusion
Recap of what was achieved through the integration of Plaid into a Flutter app.
Encouragement to explore further use cases and features of Plaid.
Invitation for feedback and sharing of personal experiences with Plaid integration.
Additional Resources
Links to Plaid’s official documentation.
Recommended tutorials and articles for deeper learning.
Community forums and support channels for developers.
Plaid is a cornerstone of the fintech ecosystem, enabling developers to build secure and seamless connections between their applications and users' financial accounts. Integrating Plaid into a Flutter app unlocks a variety of features such as bank account linking, transaction retrieval, and account balance access, making it an indispensable tool for fintech developers.
In this guide, we aim to walk you through the process of integrating Plaid into a Flutter app. By the end, you will have successfully implemented Plaid’s API for accessing financial data, ready to power your fintech solution.
Understanding Plaid
What is Plaid?
Plaid is a financial technology service that provides a secure bridge between applications and financial institutions. It allows users to link their bank accounts to apps, enabling access to financial data for a range of use cases.
Plaid API Endpoints
Transactions: Provides access to up to 24 months of user transaction data, enabling applications to offer detailed financial insights.
Accounts: Retrieves real-time account balances and details, essential for features like account verification and balance checks.
Identity: Verifies user information by accessing data from linked financial accounts, aiding in fraud prevention and compliance.
Assets: Supplies detailed reports on user assets, including account balances and holdings, useful for financial planning and lending decisions.
Investments: Offers insights into users' investment accounts, including balances, holdings, and transactions, supporting wealth management applications.
Liabilities: Retrieves information on users' liabilities, such as loans and credit card balances, assisting in comprehensive financial assessments.
Payment Initiation: Facilitates the initiation of payments directly from users' bank accounts, streamlining transactions within applications.
Income: Provides detailed information on users' income streams, supporting applications in lending and financial planning.
Monitor: Enables ongoing monitoring of user accounts for activities such as new transactions or balance changes, enhancing user engagement and security.
Use Cases for Plaid
Plaid is commonly used in applications such as:
Budgeting Apps: Utilize the Transactions endpoint to help users track expenses and manage budgets effectively.
Payment Platforms: Leverage the Accounts and Payment Initiation endpoints to verify account details and facilitate seamless transactions.
Personal Finance Tools: Access comprehensive financial data through various endpoints to provide users with holistic financial overviews and advice.
Credit Monitoring Services: Use the Liabilities and Income endpoints to assess creditworthiness and monitor financial health.
Wealth Management Platforms: Integrate the Investments and Assets endpoints to offer users detailed insights into their investment portfolios and asset distributions.
Setting Up Your Development Environment
Prerequisites
Before diving in, ensure you have the following:
Flutter SDK installed on your machine.
An integrated development environment (IDE) like VS Code or Android Studio.
A Plaid account to access API credentials.
Installing Dependencies
Install the necessary libraries by adding them to your pubspec.yaml file. For example:
dependencies:
flutter:
sdk: flutter
http: ^0.15.0
dotenv: ^3.0.0
Run Flutter Pub to install these dependencies.
Configuring Environment Variables
To securely manage your Plaid credentials, use a .env file. Add your Plaid client ID and secret to this file:
PLAID_CLIENT_ID=your_client_id
PLAID_SECRET=your_secret
PLAID_ENV=sandbox
Import and initialize the dotenv package in your project:
import 'package:flutter_dotenv/flutter_dotenv.dart';
void main() async {
await dotenv.load();
runApp(MyApp());
}
Creating a New Plaid Flutter Project
Setting Up the Project
Create a new Flutter project using the command: flutter create plaid_integration
Navigate to the project directory: cd plaid_integration
Suggested Folder Structure
Organize your project as follows:
/lib
/models
/services
/screens
/models: Define data structures for API responses.
/services: Handle API calls and business logic.
/screens: Build user interfaces.
Implementing Plaid in Your Flutter App
Step 1: Adding Plaid Dependencies
Add the Plaid SDK or equivalent package to
your Flutter app. Update pubspec.yaml to include:
plaid_flutter: ^1.0.0
Run flutter pub get to install the package.
Step 2: Authentication with Plaid
Sign up for a Plaid account and create an application.
Retrieve your client ID and secret from the Plaid dashboard.
Set these values as environment variables using the dotenv package.
Step 3: Integrating Plaid Link
Retrieving Financial Data
Accessing Transactions
Use the public token from Plaid Link to exchange for an access token via the /item/public_token/exchange endpoint.
Retrieve transaction data with an API call: final response = await http.post( Uri.parse("https://sandbox.plaid.com/transactions/get"), headers: {"Content-Type": "application/json"}, body: jsonEncode({ "client_id": dotenv.env['PLAID_CLIENT_ID'], "secret": dotenv.env['PLAID_SECRET'], "access_token": accessToken, }), ); if (response.statusCode == 200) { final data = jsonDecode(response.body); print("Transactions: ${data['transactions']}"); } else { print("Error: ${response.body}"); }
Accessing Account Information
Fetch account details similarly using the /accounts/balance/get endpoint. Parse the JSON response to display balance and account information in your app.
Error Handling and Troubleshooting
Common Issues
Invalid credentials: Ensure environment variables are correctly set.
API timeouts: Implement retry logic for API requests.
Best Practices
Log errors for debugging.
Display user-friendly error messages.
Resources
Refer to the Plaid documentation.
Join developer forums for additional support.